GDScript
The Mirror provides access to the power of Godot's GDScript. This is like normal GDScript, but supports multiple scripts per object, and includes access to The Mirror's API.
The Mirror provides GDScript primarily for existing Godot users, and as such, The Mirror's documentation will not cover every aspect of the GDScript language. For more information on GDScript, please refer to the Godot documentation.
Mirror-Flavored GDScript
You may use functions and variables just like you would in normal GDScript. As a part of The Mirror's support for multiple scripts per object, you can use members of SpaceObject the same way you use inherited members in Godot. For example, print(position)
will print a SpaceObject's position. In this way, your GDScript code behaves as if it were directly in a script attached to the SpaceObject, without actually being attached to it.
If you need to refer to the object the script is attached to, use the target_object
variable. The self
variable refers to the script itself. The target_object
variable can either be a SpaceObject or the global scripts singleton.
You must not write class_name
or extends
in your script. The Mirror's support for multiple scripts per object means that scripts act more like "components", so they do not extend the object they are attached to.
Entries
Entry points can be added to a script by clicking the "Add Entry" button in the script editor. This is the same as the "Entry" section of the "Add Script Block" menu in visual scripting. These use Godot signals under the hood, but The Mirror handles the connection for you.

When an entry is connected, it will show a green arrow icon to the left of the function signature. If you do not see a green arrow, then the entry is not connected, and you may need to add it from the Add Entry menu again. To delete an entry, erase the function text, and then the signal will automatically be disconnected.
The callback functions _ready
, _process
, and _physics_process
are available, however others such as the input callbacks are not available. When detected, they will show a blue arrow. These are provided for convenience and compatibility with regular GDScript. Generally, the entry points added through "Add Entry" are the preferred way to receive signals from The Mirror.
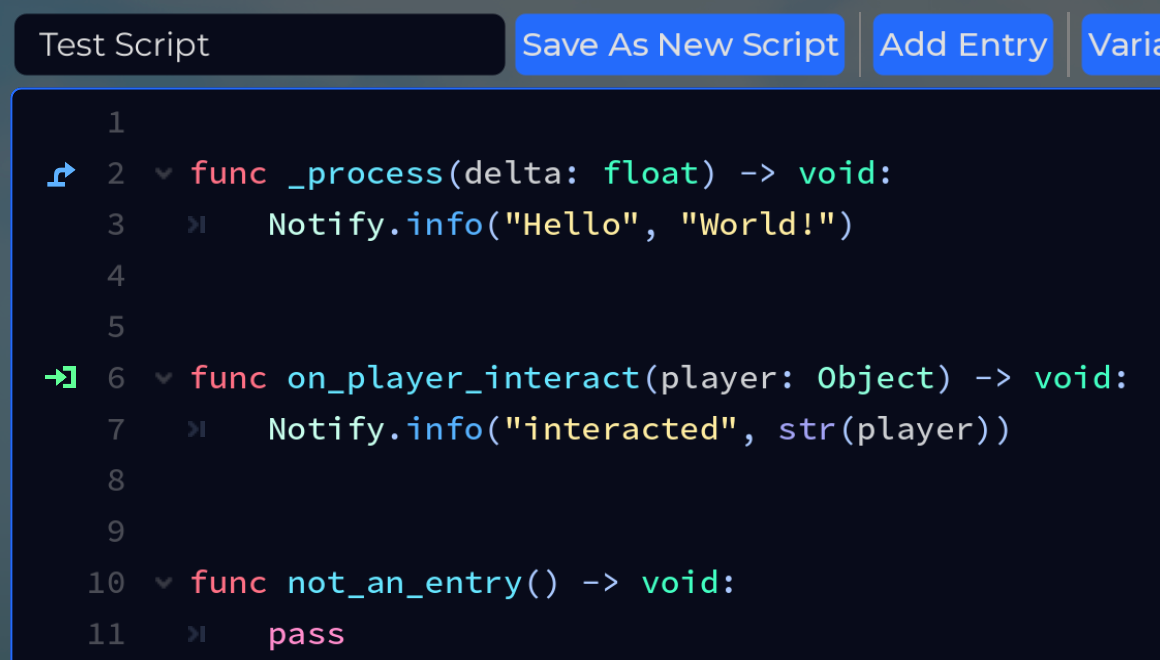
API
You can print to the notification area using Notify.info(title, message)
. You can also use Notify.success
, Notify.warning
, and Notify.error
.
Many helper functions for functionality present in visual scripting are available via the Mirror
singleton. For example, functions for accessing and modifying variables are here.
Example: Counter
Here is a simple script that counts every frame and prints to the notification area with Notify.info
.
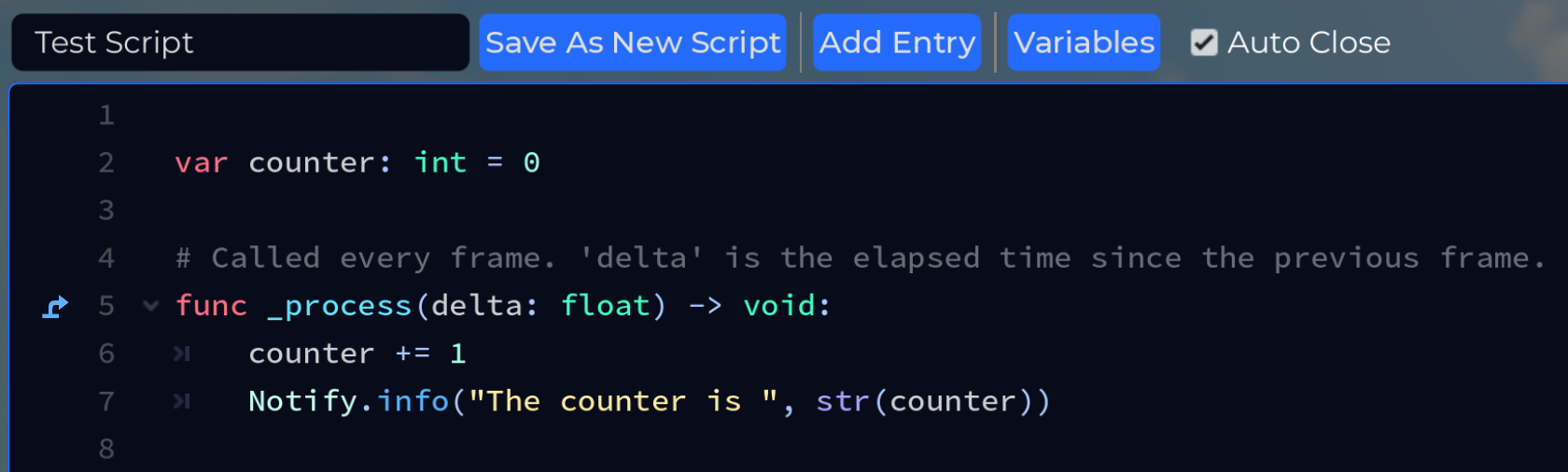
However, the above uses a member variable. This is useful for its own reasons, but it is not accessible by other scripts, it is not persistent and so will reset if the server restarts, and will reset any time you change the script. If you want a variable that is persistent, or something that can share data between scripts, you can use an object variable. You can use the get_object_variable
and set_object_variable
functions to access these.
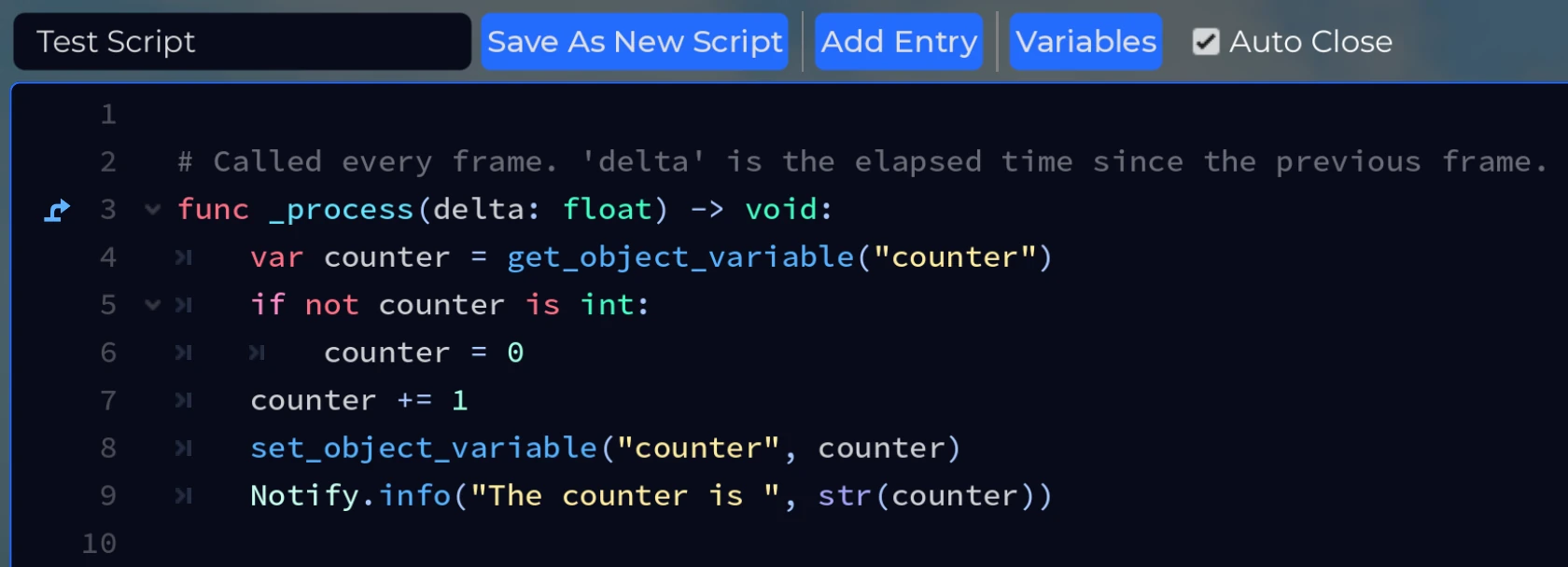
The Mirror's object and global variables behave the same way in GDScript as they do in visual scripting.
Example: Has Red Key
Aside from setting variables on yourself, you can also use the Mirror.get_object_variable
and Mirror.set_object_variable
functions to access variables on other objects. These functions work on any node, including SpaceObjects, model subnodes, and even players. By itself, get_object_variable(...)
is a shorthand for Mirror.get_object_variable(target_object, ...)
. You can also use Mirror.get_global_variable
and Mirror.set_global_variable
to access global variables.
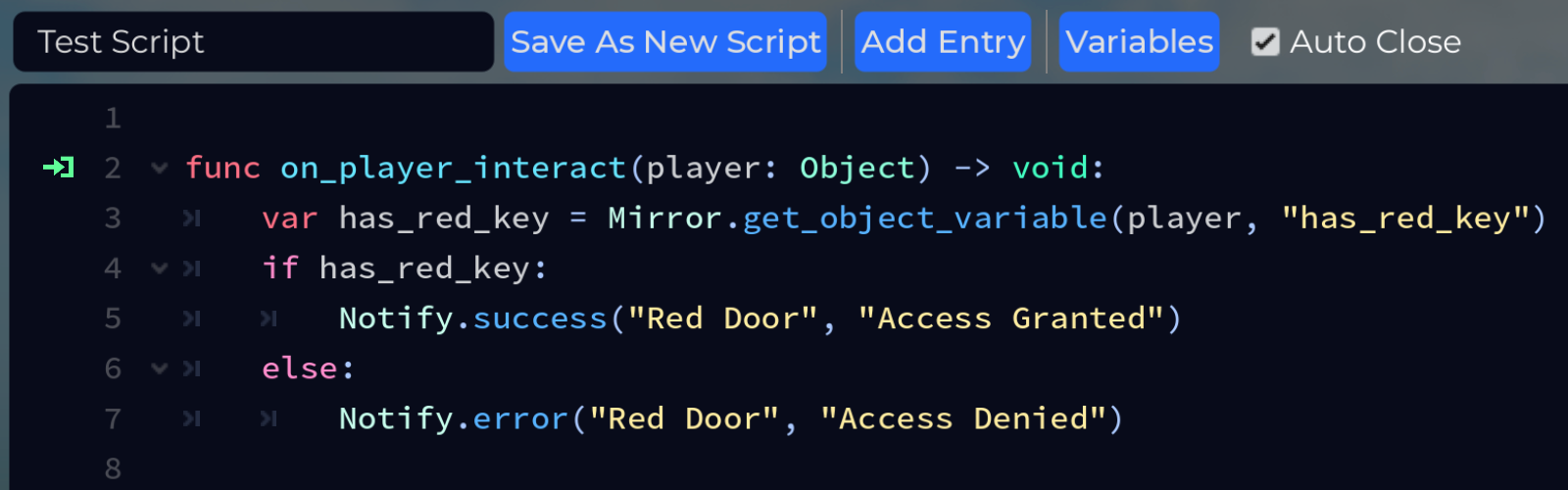
By default, the above script will show an "Access Denied" message if the "has_red_key"
variable is null or false, indicating that the player does not have the red key. If another script granted the player the red key by setting "has_red_key"
to true, then it will show an "Access Granted" message instead.
Note that variable names are String
, not StringName
. This is because The Mirror's variables support JSON paths separated by either dots or forward slashes, and so are not limited to simple names. For example, "a/b"
and "a.b"
both refer to the same variable "b"
inside of a Dictionary
variable "a"
. If desired, you could instead use the variable path "door_keys/has_red"
to store the variable "has_red"
inside of a Dictionary
variable "door_keys"
.